Trade Widget SDK
Seamless crypto trading
With our Trade SDK you can Integrate our Trading Widget directly into your web application through simple code. You can adjust the colors on the Trade Widget according to your branding and styling, and we take care of everything else.
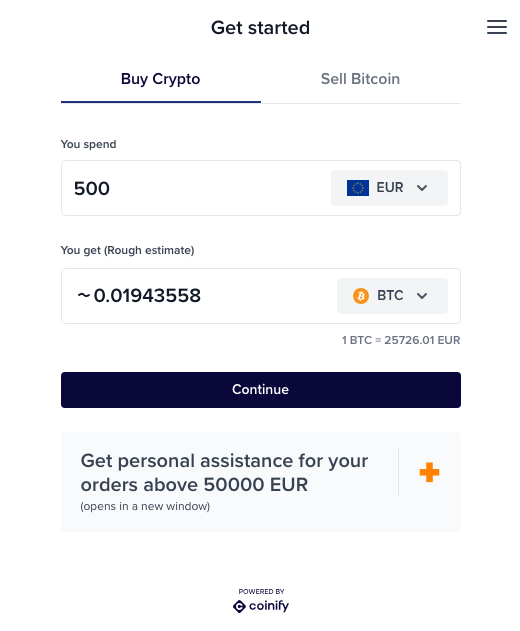
Trade Widget UI example
Trade Widget SDK
In this guide we will walk you through the process of integrating our SDK to your web application. The client-side code uses our Trade Widget with multiple options to complete the trades.
Your customers can select which cryptocurrency they want to buy and choose one of the options to complete the payment for the specified amount and cryptocurrency. On the other hand, when selling, the customers can either scan the QR code or copy the details to their crypto-wallet and execute the blockchain transaction accordingly in order to successfully complete the Sell trade and receive the FIAT counter value of the cryptocurrency they've sold.
Web app integration
- Provide your
partnerId
andpartnerName
. - Render the Trade Widget inside your web application.
- You can load the SDK from this URL on sandbox:
https://trade-ui.sandbox.coinify.com/widget/sdk/v1/trade.js
. - Use any of the optional supported parameters (listed below) to customise the widget look and behaviour according to your needs.
Here's an example HTML for loading the Trade Widget in sandbox environment
<!DOCTYPE html>
<html lang="en">
<head>
<title>Coinify SDK example</title>
<style>
body {
font-family: Helvetica, Arial, sans-serif;
margin: 0; background-color: #f9fbfc; overflow: clip;
text-align: center;
}
.container {
display: flex; justify-content: center; align-items: center;
height: 80vh; width: 90%; margin: 5vh 5vw;
border: 1px solid #BFBFBF; background-color: white; box-shadow: 10px 10px 5px #aaaaaa;
}
</style>
<script src="https://trade-ui.sandbox.coinify.com/widget/sdk/v1/trade.js"></script>
</head>
<body>
<h1 style="">Trade SDK sample integration</h1>
<div id="container-id" class="container"></div>
<script type="text/javascript">
const widget = Coinify
.Trade({
// required partner configuration
partnerId: 'your-partner-id', // string
partnerName: 'your-partner-name', // string
})
// optional, register events emitted from the widget
.on('trade.trade-prepared', (e) => console.log('trade prepared', e))
.on('trade.trade-created', (e) => console.log('trade created', e))
.on('trade.trade-placed', (e) => console.log('trade placed', e))
// render
.render({
containerId: 'container-id', // element id to render
parameters: {
// optional parameters defining widget behaviour
// primaryColor: 'green', // color code
// noMenu: false, // boolean
// noSignup: 'corporate', // 'corporate' | 'individual' | 'corporate' | boolean
// confirmMessages: true, // boolean
//
// refreshToken: 'your-token', // string
// targetPage: 'sell', // 'login' | 'signup' | 'signup-corporate' | 'buy' | 'sell' | 'trade-history'
// partnerContext: { my: 'context', is: true }, // object literal
//
// cryptoCurrencies: ['BTC', 'ETH'], // string[],
// fiatCurrencies: ['USD', 'DKK'], // string[],
// transferInMedia: ['bank', 'blockchain'], // <'bank' | 'card' | 'blockchain'>[],
// transferOutMedia: ['blockchain', 'bank'], // <'bank' | 'card' | 'blockchain'>[]
//
// buyAmount: 123.45, // number
// isBuyAmountFixed:true // boolean
// isSellAmountFixed:true // boolean
// sellAmount: 0.01235, // number
// defaultCryptoCurrency: 'ETH', // string
// defaultFiatCurrency: 'DKK', // string
//
// address: { BTC: 'some-BTC-address', ETH: 'some-ETH-address' }, // string | Record<string, string>
// addressSignature: 'abc', // string | Record<string, string>
// memo: 'some-memo' // string | Record<string, string>
}
});
// optional, sending messages to the widget
const changeContext = () => {
widget.send('settings.partner-context-changed', {
partnerContext: {
some: 'partner context',
date: new Date().toISOString()
}
});
};
const confirmTradePrepared = (confirmed) => {
widget.send('trade.confirm-trade-prepared', { confirmed });
};
const confirmTradeCreated = (confirmed) => {
widget.send('trade.confirm-trade-created', { confirmed });
};
</script>
<div style="margin: 0 5vw">
Trigger a message to be sent:
<Button onclick="changeContext()" type="button">Change context</Button>
<Button onclick="confirmTradePrepared(true)" type="button">Accept trade prepared</Button>
<Button onclick="confirmTradePrepared(false)" type="button">Reject trade prepared</Button>
<Button onclick="confirmTradeCreated(true)" type="button">Accept trade created</Button>
<Button onclick="confirmTradeCreated(false)" type="button">Reject trade created</Button>
</div>
</body>
</html>
In the following table you can find the description and usage for each of the Trade Widget parameters:
Parameter | Description |
---|---|
partnerId | (Mandatory) Unique partner ID (UUID) as provided by Coinify during the Partner onboarding process. |
partnerName | Your company name to be displayed in the widget as the partner name. Currently used only when displaying partner fee, if enabled. Defaults to Partner . |
primaryColor | Color of the primary button. Accepts: named value e.g. blue , hash value e.g. #00ff00, or rgba(0,255,0,1) . |
noMenu | Enables removing the top-right burger menu. Useful when e.g. the Trusted Email Validation flow is set-up and the users are Signed-up/Logged-in automatically. |
noSignup | Defines whether to support signup through the widget. Note the negation! Available values are false (allows signup for corporate & individuals), true (allows no signup), corporate (allow signup only for individual traders) and individual (allow signup only for corporate traders). Not using this parameter defaults to allowing signup for both individual and corporate traders. |
confirmMessages | Defines if external confirmation of trade messages is required. When provided the widget will await confirmation messages from hosting window before continuing flow. See details on how to use messages. |
refreshToken | Used for custom onboarding flows. The refreshToken can be obtained by calling one of the auth endpoints. The refreshToken should be URL encoded so that the browsers interprets it correctly. |
targetPage | Defines a target landing page other than the default. Available values are login , signup , signup-corporate , buy , sell and trade-history . |
partnerContext | Free context for partners to receive in webhooks, can be stringified JSON as well. Partner context may also be provided using [settings.partner-context-changed](https://developer.coinify.com/apidoc/trade/#widget-events-settings-partner-context-changed) |
cryptoCurrencies | Comma separated list of crypto currencies to support in the widget. Will include only the specified crypto currencies from those supported by default. You can find the list of supported cryptocurrencies via this API endpoint. E.g. cryptoCurrencies=['BTC', 'ETH', 'XLM'] |
fiatCurrencies | Comma separated list of fiat currencies to support in the widget. Will include only the specified fiat currencies from those supported by default. You can find the list of supported cryptocurrencies via this API endpoint. E.g. fiatCurrencies=['USD','EUR'] . |
transferInMedia | Specifies the inbound transaction type. In combination with the transferOutMedia determines the trade type (Buy or Sell). See all transfer media types and descriptions. Available values: card , bank , blockchain . See details on how to use the parameter here. |
transferOutMedia | Specifies the outbound transaction type. See all transfer media types and descriptions. Available: bank , blockchain . See details on how to use the parameter here. |
buyAmount | Defines initial amount on Buy quotes. Note, often used with targetPage , defaultCryptoCurrency , and defaultFiatCurrency parameters. |
isBuyAmountFixed | If set to true , the specified buyAmount is locked and cannot be changed from the Trade Widget UI. Accepts true or false values. |
sellAmount | Defines initial amount on Sell quotes. Note, often used with targetPage , defaultCryptoCurrency , and defaultFiatCurrency parameters. |
isSellAmountFixed | If set to true , the specified sellAmount is locked and cannot be changed from the Trade Widget UI. Accepts true or false values. |
defaultCryptoCurrency | Preselects the cryptocurrency initially selected on Trade Widget's Buy/Sell quotes if multiple crypto currencies are available. |
defaultFiatCurrency | Preselects the cryptocurrency initially selected on Trade Widget's Buy/Sell quotes if multiple crypto currencies are available. |
address | Sets the receiving wallet address when buying cryptocurrencies. Also used to set the refund wallet address when selling cryptocurrencies in case the sell trade cannot be completed. See details on how to use address. |
addressSignature | Provides HMAC-SHA-256 signature for address. Only used if a shared secret has been established with Coinify. See details on how to use address signature. |
memo | An optional memo/destination tag accepted by some crypto currencies. Memo follows the same format as the address and addressSignature parameters. |