This section describes the trade object as well as the endpoints that allow you to create, query and manipulate trades:
- See all payment methods for trades
- Request trade price quote
- Get price quote details
- See payment methods for price quote
- Create trade
- Get trade information
- Cancel trade
The following sections describe the trade object and continue to describe each of the endpoints in greater detail.
Trade object
Example
awaiting_transfer_in
trade object buying 2.41526674 BTC for 1,000.00 USD
{
"id": 113475347,
"traderId": 754035,
"traderEmail": "customer@coinify.com",
"state": "awaiting_transfer_in",
"inCurrency": "USD",
"outCurrency": "BTC",
"inAmount": 1000.00,
"outAmountExpected": 2.41526674,
"transferIn": {
"currency": "USD",
"sendAmount": 1000.00,
"receiveAmount": 1000.00,
"medium": "card",
"details": {
"redirectUrl": "https://provider.com/payment/d3aab081-7c5b-4ddb-b28b-c82cc8642a18"
}
},
"transferOut": {
"currency": "BTC",
"medium": "blockchain",
"sendAmount": 2.41526674,
"receiveAmount": 2.41526674,
"details": {
"account": "1A1zP1eP5QGefi2DMPTfTL5SLmv7DivfNa"
}
},
"quoteExpireTime": "2016-04-01T12:38:19Z",
"updateTime": "2016-04-01T12:27:36Z",
"createTime": "2016-04-01T12:23:19Z"
}
Example
completed
trade object buying 2.41551728 BTC for 1,000.00 USD
{
"id": 113475347,
"traderId": 754035,
"traderEmail": "customer@coinify.com",
"state": "completed",
"inCurrency": "USD",
"outCurrency": "BTC",
"inAmount": 1000.00,
"outAmount": 2.41551728,
"transferIn": {
"currency": "USD",
"sendAmount": 1000.00,
"receiveAmount": 1000.00,
"medium": "card",
"details": {
"redirectUrl": "https://provider.com/payment/d3aab081-7c5b-4ddb-b28b-c82cc8642a18"
}
},
"transferOut": {
"currency": "BTC",
"medium": "blockchain",
"sendAmount": 2.41526674,
"receiveAmount": 2.41526674,
"details": {
// Trader's bitcoin address that has received the 2.41551728 BTC
"account": "1A1zP1eP5QGefi2DMPTfTL5SLmv7DivfNa",
// The BTC transaction that sent out the BTC to the above address
"transaction": "000000000019d6689c085ae165831e934ff763ae46a2a6c172b3f1b60a8ce26f"
}
},
"updateTime": "2016-04-01T12:27:36Z",
"createTime": "2016-04-01T12:23:19Z"
}
Example
completed
trade object selling 2.41551728 BTC for 1,000.00 USD
{
"id": 113475348,
"traderId": 754035,
"traderEmail": "customer@coinify.com",
"state": "completed",
"inCurrency": "BTC",
"outCurrency": "USD",
"inAmount": 2.41551728,
"outAmount": 1000.00,
"transferIn": {
"currency": "BTC",
"sendAmount": 2.41551728,
"receiveAmount": 2.41551728,
"medium": "blockchain",
"details": {
// Coinify's bitcoin address to where the trader sent the 2.41551728 BTC
"account": "1A1zP1eP5QGefi2DMPTfTL5SLmv7DivfNa",
"paymentUri": "bitcoin:1A1zP1eP5QGefi2DMPTfTL5SLmv7DivfNa?amount=2.41551728",
// The BTC transaction that sent out the BTC to the above address
"transaction": "000000000019d6689c085ae165831e934ff763ae46a2a6c172b3f1b60a8ce26f"
}
},
"transferOut": {
"currency": "USD",
"sendAmount": 1000.00,
"receiveAmount": 1000.00,
"medium": "bank",
"mediumReceiveAccountId": 12345 // Reference to the traders bank account
},
"updateTime": "2016-04-01T12:27:36Z",
"createTime": "2016-04-01T12:23:19Z"
}
The following table defines the fields of a trade object:
Key | Type | Description |
---|---|---|
id | Integer | Unique ID for this trade |
traderId | Integer | Reference to the trader that created the trade |
traderEmail | String | Email address of trader that created the trade |
state | Trade state | The current state of the trade |
inCurrency | String | Currency (ISO 4217) denominating inAmount . |
outCurrency | String | Currency (ISO 4217) denominating outAmount or outAmountExpected . |
inAmount | Float | The amount of inAmount that this trade covers. Is always positive. |
outAmount | Float | (Optional) The amount of outCurrency that this trade resulted in. Is always positive. NOTE: This field is only defined if the state of the trade is completed . For all other states, see the outAmountExpected field. |
outAmountExpected | Float | The amount of outCurrency that this trade is expected to result in. Is always positive. |
transferIn | Transfer object | Object describing how we (Coinify) will receive/have received the money to fund the trade. |
transferOut | Transfer object | Object describing how we (Coinify) will send/have sent the result of the trade back to the trader. |
quoteExpireTime | ISO 8601 time | (Optional) The time when the price quote underlying this trade expires. NOTE: This field is only defined in the following states: awaiting_transfer_in , processing , reviewing , payment_authorized . |
updateTime | ISO 8601 time | The time when the trade was last updated. |
createTime | ISO 8601 time | Timestamp for when this trade was first created |
Trade states
The following table lists all possible states of a trade (the state
property):
State | Description |
---|---|
awaiting_transfer_in | (Starting state) Trade was successfully created, and is waiting for trader's payment |
payment_authorized | (Only if transferIn.medium = 'card') We have reserved trade.inAmount on customers card. Followed immediately by processing if trader has performed Mandatory onboarding steps and the quote is not expired. |
processing | Trade has received the trader's payment, and we are processing the trade. |
completed | (Ending state) Trade completed successfully. |
cancelled | (Ending state) Trade cancelled. |
rejected | (Ending state) Trade was rejected. |
expired | (Ending state) Trade expired before it completed. |
completed
state, there is also a nearly-identical completed_test
state, which is used instead of completed
if the trade was paid for with a test payment. See the section on testing for more information.
The states, as well as the possible transitions between them, are visualized in this diagram:
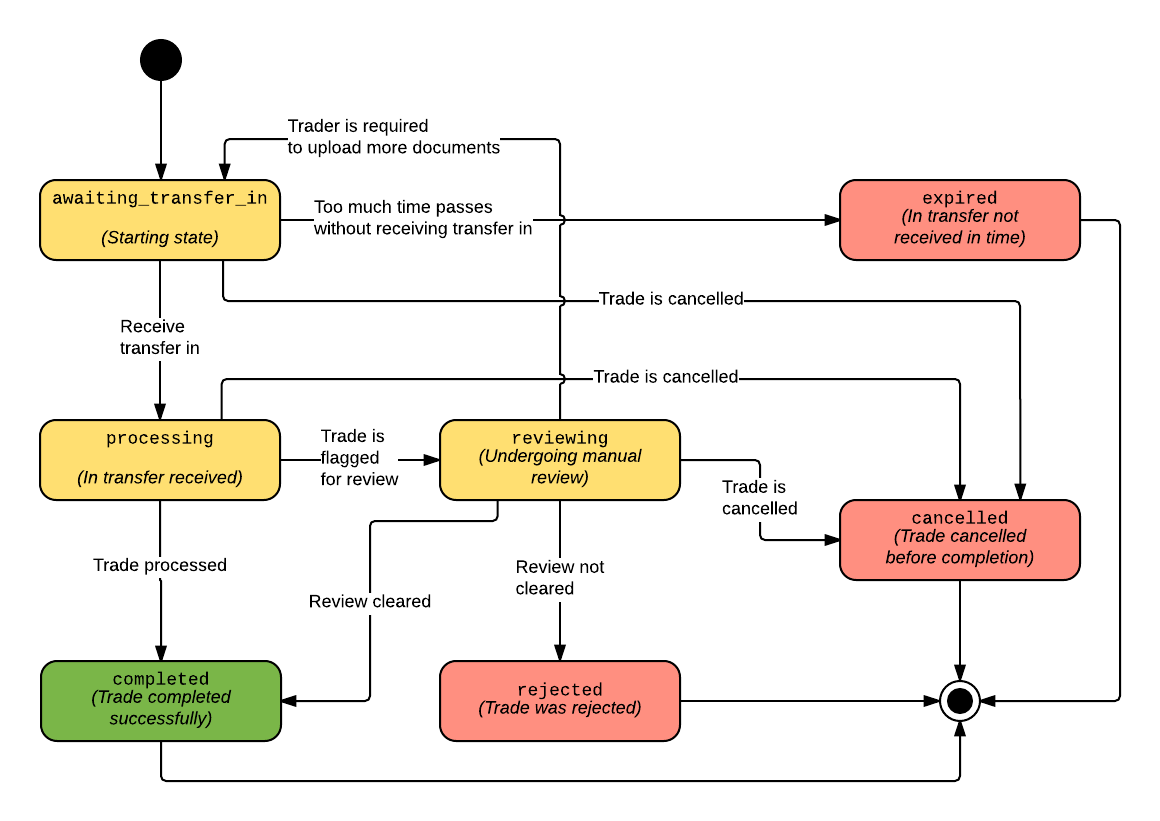
Transfer object
Each trade has two transfer objects - transferIn
and transferOut
- which contain details about how Coinify will receive money from the trader, and how Coinify will send money back to the trader, respectively.
Each transfer object contains the following keys:
Key | Type | Description |
---|---|---|
currency | String | Currency (ISO 4217) that this transfer is/will be denominated in. |
sendAmount | Float | Amount that is/will be sent to this transfer. Denominated in currency . Is always positive. |
receiveAmount | Float | Amount that this transfer will result in. Denominated in currency . Is always positive and equal to or smaller then sendAmount . The difference between the sendAmount and the receiveAmount will be the fee of the transfer. |
medium | String | Transfer medium. The medium transferring the currency. |
mediumReceiveAccountId | Integer | Reference to our internal accounts. Note at the moment we only have internal bank accounts. |
details | Medium details | Information relevant for this medium |
Transfer media
Medium | Description |
---|---|
blockchain | Blockchain - Transfer on a public blockchain, such as Bitcoin. NOTE: The currency field of the transfer object will determine which blockchain currency. |
card | Card - Card payment. |
bank | Bank - Bank account payment. If transfer is incoming we sent our own bank details as the details object. And if transfer is outgoing its the details of the traders bank account. |
Blockchain
Details object
Key | Type | Description |
---|---|---|
account | String | Account receiving the money in this transfer (blockchain address). |
accountSignature | String | HMAC-SHA-256 signature signing the account value. Only defined if a shared secret has been established with Coinify. |
memo | String | Optional memo for currencies where memo/destination tag is supported. |
transaction | String | Transaction sending the money in this transfer. Only defined if money has been sent. |
refundAccount | String | Account to refund the money in this transfer in case of failure. Only defined if currency != 'BTC' . |
refundAccountSignature | String | HMAC-SHA-256 signature signing the refundAccount value. Only defined if refundAccount is defined and a shared secret has been established with Coinify. |
paymentUri | String | Payment URI scheme to generate QR code. |
Card
Details object
Below, a description of the request/response objects for creating a trade.
Request object
Key | Type | Description |
---|---|---|
returnUrl | String | The return URL to which the user to be sent back after the payment has been created. Can be provided when creating a trade. |
Reponse object
Key | Type | Description |
---|---|---|
redirectUrl | String | Source URL to process the payment. |
returnUrl | String | The return URL to which the user to be sent back after the payment has been created. |
Bank
Details object
Details for the bank transfer is populated automatically for incoming transfers, where the trader needs to send money to our account. If the transfer is outgoing, you must specify the accountId
for the traders bank account to use.
Key | Type | Description |
---|---|---|
referenceText | String | Text that the bank transfer must contain in order for Coinify to correctly register what trade it concerns. |
account | Object | Object with additional information about the bank account. |
→currency | String | Currency of the bank account. |
→type | Bank account type | Type of the bank account |
→bic | String | For sepa and international its the SWIFT / BIC number and for danish accounts its the REG number. Optional in case of domestic bank tansfers. |
→number | String | For sepa and international it's the IBAN (International Bank Account Number). For danish accounts, it's the BBAN (Basic Bank Account Number). Optional in case of domestic bank transfers. |
→domesticAccountNumber | String | Account Number for domestic transfers. (Optional) |
→regNo | String | Reg. No. used for some domestic transfers. (Optional) |
→routingCode | String | Routing Code used for some domestic transfers. (Optional) |
→sortCode | String | Sort Code used for some domestic transfers. (Optional) |
→bsb | String | BSB used for some domestic transfers. (Optional) |
bank | Object | Object with additional information about the bank. |
→name | String | Name of the bank. |
→address | Object | Object with information about the address of the bank. |
→street | String | Street address. |
→zipcode | String | Zip/Postal code. |
→city | String | City. |
→state | String | State. |
→country | String | ISO 3166-1 alpha-2 country code |
holder | Object | Object with additional information about the bank account holder. |
→name | String | Name of the bank account holder. |
→address | Object | Object with information about the address of the account holder. |
→street | String | Street address. |
→zipcode | String | Zip/Postal code. |
→city | String | City. |
→state | String | State. |
→country | String | ISO 3166-1 alpha-2 country code |
Payment methods
This section contains information about different methods to buy/sell blockchain currencies.
The currently available payment methods are:
- Buy crypto currency with bank transfer - (in) bank, (out) blockchain
- Buy crypto currency with card transfer - (in) card, (out) blockchain
- Sell bitcoins to bank transfer - (in) blockchain, (out) bank
Payment method object
The object represents a single payment method, containing the information about how and with what currencies the money
transfer can and should be performed, and optionally the fees that are going to be applied to the amounts.
Key | Type | Description |
---|---|---|
inMedium | String | The medium for the in transfer of the trade - by what way the customer pays |
outMedium | String | The medium for the out transfer of the trade - what way the customer receives funds |
inCurrencies | List | The possible currencies in which the incoming amount can be made, optionally filtered by request arguments |
outCurrencies | List | The possible currencies in which the outgoing amount can be made, optionally filtered by request arguments |
inFixedFees | Object | Object of inCurrencies and fixed fees for each currency for the in transfer. |
inPercentageFee | Float | Percentage fee for the in transfer. |
minimumInFees | Object | Minimum fee for the in transfer. |
outFixedFees | Object | Object of outCurrencies and fixed fees for each currency for the out transfer. |
outPercentageFee | Float | Percentage fee for the out transfer. |
minimumOutFees | Object | Minimum fee for the out transfer. |
minimumInAmounts | Object | Object of inCurrencies and the minimum limit for each. |
limitInAmounts | Object | Object of inCurrencies and the trader's current limit for each currency, based on the current limits of the trader. Note: Only included for authenticated requests. |
canTrade | Boolean | Can this trader create new trades for this payment method? Note: Only included for authenticated requests. If inCurrency , inAmount , outCurrency and outAmount are all provided in request, this value determines if a trade with the specific amounts/currencies can be made. Otherwise, this value determines if any trade can be made with this payment method. |
cannotTradeReasons | List | (Optional) List of reason objects why the trader cannot create new trades (why canTrade is false ). Note: Only included for authenticated requests if canTrade is false . |
inCurrency | String | (Optional, if inCurrency parameter provided) Echo of inCurrency parameter. |
inAmount | Float | (Optional, if inAmount parameter provided) Echo of inAmount parameter. |
outCurrency | String | (Optional, if outCurrency parameter provided) Echo of outCurrency parameter. |
outAmount | Float | (Optional, if outAmount parameter provided) Echo of outAmount parameter. |
cannotTradeReason
object
cannotTradeReason
objectThis object represents a reason for why a trader cannot create a trade with a given payment method. The only field that is always present in the object is the reasonCode
. Depending on the value of the reasonCode
, other reason-specific fields may be present in the object.
Key | Type | Description |
---|---|---|
reasonCode | String | Machine-readable code for the reason why the trade cannot create a trade. See Possible reasonCode s for a list of possible values |
Possible reasonCode
s
reasonCode
sreasonCode value | Description | Extra fields |
---|---|---|
forced_delay | Trader must wait until a specific time before creating trade | delayEnd (string): ISO-8601 timestamp for when the delay is over. |
trade_in_progress | Trader must wait until a specific trade has completed | tradeId (integer): ID of trade that must be completed |
limits_exceeded | Creating trade would exceed the trader's limits | None |
country_not_supported | Trading is not currently supported in the trader's country. See supported countries for querying a partner's supported countries. | None |
List payment methods
Example response for
GET /trades/payment-methods
(authenticated)
[
{
"inMedium": "bank",
"outMedium": "blockchain",
"inCurrencies": ["DKK", "EUR", "USD", "GBP", "CHF"],
"outCurrencies": ["BTC", "ETH", "BSV", "BCH", "XLM", "DASH", "NANO"],
"inFixedFees": {
"DKK": 0,
"EUR": 0,
"USD": 0,
"GBP": 0,
"CHF": 0
},
"inPercentageFee": 0.75,
"minimumInFees": {
"DKK": 49.00,
"EUR": 4.99,
"USD": 4.99,
"GBP": 4.99,
"CHF": 4.99
},
"outFixedFees": {
"BTC": 0.0002,
"ETH": 0,
"BSV": 0,
"BCH": 0,
"XLM": 0,
"DASH": 0,
"NANO": 0
},
"outPercentageFee": 0,
"minimumInAmounts": {
"DKK": 933.38,
"EUR": 125,
"USD": 140.17,
"GBP": 111.35,
"CHF": 140.08
},
"limitInAmounts": {
"DKK": 7500.86,
"EUR": 1000.00,
"USD": 1200.50,
"GBP": 8000.00,
"CHF": 800.00
},
"canTrade": true
},
{
"inMedium": "blockchain",
"outMedium": "bank",
"inCurrencies": ["BTC", "ETH", "BSV", "BCH", "XLM", "DASH", "NANO"],
"outCurrencies": ["DKK", "EUR", "USD", "GBP", "CHF"],
"inFixedFees": {
"BTC": 0,
"ETH": 0,
"BSV": 0,
"BCH": 0,
"XLM": 0,
"DASH": 0,
"NANO": 0
},
"inPercentageFee": 0,
"outFixedFees": {
"DKK": 40.00,
"EUR": 5.40,
"USD": 6.10,
"GBP": 3.70,
"CHF": 4.90
},
"minimumOutFees": {
"DKK": 49.00,
"EUR": 4.99,
"USD": 4.99,
"GBP": 4.99,
"CHF": 4.99
},
"outPercentageFee": 1,
"minimumInAmounts": {
"BTC": 0.01530498,
"ETH": 0.514718127075,
"BSV": 0.65349743,
"BCH": 0.32362399,
"XLM": 1077.1290499,
"DASH": 1.77,
"NANO": 231.41
},
"limitInAmounts": {
"BTC": 0.1224398,
"ETH": 4.117745016597,
"BSV": 5.2279794,
"BCH": 2.58899193,
"XLM": 8617.0323993,
"DASH": 15.2,
"NANO": 1249.23
},
"canTrade": true
},
{
"inMedium": "card",
"outMedium": "blockchain",
"inCurrencies": ["DKK", "EUR", "USD", "GBP", "CHF"],
"outCurrencies": ["BTC", "ETH", "BSV", "BCH", "XLM", "DASH", "NANO"],
"inFixedFees": {
"DKK": 0,
"EUR": 0,
"USD": 0,
"GBP": 0,
"CHF": 0
},
"inPercentageFee": 3.5,
"minimumInFees": {
"DKK": 49.00,
"EUR": 4.99,
"USD": 4.99,
"GBP": 4.99,
"CHF": 4.99
},
"outFixedFees": {
"BTC": 0.0002,
"ETH": 0,
"BSV": 0,
"BCH": 0,
"XLM": 0,
"DASH": 0,
"NANO": 0
},
"outPercentageFee": 0,
"minimumInAmounts": {
"DKK": 149.34,
"EUR": 20,
"USD": 22.43,
"GBP": 17.82,
"CHF": 22.41
},
"limitInAmounts": {
"DKK": 746.71,
"EUR": 100,
"USD": 112.14,
"GBP": 89.08,
"CHF": 112.06
},
"canTrade": false,
"cannotTradeReasons": [
{
"reasonCode": "forced_delay",
"delayEnd": "2016-04-01T12:27:36Z"
}
]
}
]
GET https://app-api.coinify.com/trades/payment-methods
This endpoint returns a list of payment methods objects, available for creating and paying for trades.
If you want know which network/chain does a particular supported cryptocurrency belong to, please find the list here.
Response
HTTP Response code | Error code | JSON data |
---|---|---|
200 OK | Success, response as shown to the right. | |
400 Bad request | invalid_argument | Error, interpreting the request (wrong input values for query parameters). |
500 Internal error | internal_error | Error, an internal error happened. |
Request trade price quote
Example request for
POST /trades/quote
// This request is saying: "I want to buy BTC for 1,000.00 USD. How much BTC will I get?"
{
"baseCurrency": "USD",
"quoteCurrency": "BTC",
"baseAmount": -1000.00
}
Example response for
POST /trades/quote
// This response is saying "We'll give you 2.41551728 BTC for 1,000.00 USD"
{
"id": 123456,
"baseCurrency": "USD",
"quoteCurrency": "BTC",
"baseAmount": -1000.00,
"quoteAmount": 2.41551728,
"issueTime": "2016-04-01T11:47:24Z",
"expiryTime": "2016-04-01T12:02:24Z"
}
POST https://app-api.coinify.com/trades/quote
Before actually creating a trade, you (as a trader) have the option of getting a price quote (i.e. an offer for a specific exchange rate) that is valid for a short period of time. When creating a trade you can reference a price quote and trade using the rate given in the quote.
Currently, when purchasing BTC and paying with a credit card, the price quote is valid for 15 minutes after being issued. For other currencies and payment methods the quote is only indicative. The price quote is only valid for the trader that requested it. You will receive an error if you try to create a trade using a price quote requested by another trader.Request object
The parameters in the request object are as follows:
Parameter | Type | Default | Description |
---|---|---|---|
baseCurrency | String | Required | Relative to what currency (ISO 4217) do you want a price quote? Denominates baseAmount . |
quoteCurrency | String | Required | What currency (ISO 4217) do you want the price quote in? |
baseAmount | Float | Required | Amount to get a price quote for. Denominated in baseCurrency . |
transferIn | Object | Empty object | An object describing how Coinify will receive the money for this transfer. |
→ medium | String | null | Transfer medium |
transferOut | Object | Empty object | An object describing how Coinify will send the result of the trade to the trader. |
→ medium | String | null | Transfer medium |
Request object examples
The following table exemplifies how baseCurrency
and quoteCurrency
should be interpreted.
baseCurrency | quoteCurrency | baseAmount | Interpretation |
---|---|---|---|
"USD" | "BTC" | 123.45 | I want to buy 123.45 USD for BTC. |
"USD" | "BTC" | -123.45 | I want to sell 123.45 USD for BTC. |
"BTC" | "USD" | 1.5 | I want to buy 1.5 BTC for USD. |
"BTC" | "USD" | -1.5 | I want to sell 1.5 BTC for USD. |
Response object
The success response object contains the following fields:
Key | Type | Description |
---|---|---|
id | Integer | Price quote identifier. Pass this identifier to the create trade endpoint to claim the offer. Note: Not included if anonymous (un-authenticated) request. |
baseCurrency | String | Currency (ISO 4217) denominating baseAmount , the known amount. |
quoteCurrency | String | Currency (ISO 4217) denominating quoteAmount , the unknown amount. |
baseAmount | Float | How much of baseCurrency does this quote apply for? |
quoteAmount | Float | How much of quoteCurrency is this quote? This is the "result", the price quote. |
transferIn | Object | transferIn object, only returned if provided in request |
→ medium | String | Transfer medium |
→ feeAmount | Float | Transfer fee (Will be added on the trade) |
→ currency | String | Currency |
transferOut | Object | transferOut object, only returned if provided in request |
→ medium | String | Transfer medium |
→ feeAmount | Float | Transfer fee (Will be added on the trade) |
→ currency | String | Currency |
issueTime | ISO 8601 time | Timestamp for when this price quote was issued. |
expiryTime | ISO 8601 time | Timestamp for when this price quote expires. |
Response
HTTP Response code | JSON data |
---|---|
201 Created | Success, A Price quote created and returned object as shown to the right |
401 Unauthorized | Error, access token missing. |
Get price quote details
Example response (buy) for
GET /trades/quote/:id/details?transferInMedium=card&transferOutMedium=blockchain
{
"transferIn": {
"currency": "EUR",
"isMinimumHandlingFeeApplied": false,
"isMaximumHandlingFeeApplied": false,
"sendAmount": 103.25,
"receiveAmount": 100,
"totalFee": 3.25,
"partnerFee": 3, // Not present if partner fee is not enabled
"handlingFee": 0.25
},
"transferOut": {
"currency": "ETH",
"sendAmount": 0.009582,
"receiveAmount": 0.008582,
"networkFee": 0.001 // Not present if no network fee
},
"exchangeRate": 10436.23
}
Example response (buy) for
GET /trades/quote/:id/details?transferInMedium=card&transferOutMedium=blockchain&promoCode=12314code4321
{
"transferIn": {
"currency": "EUR",
"isMinimumHandlingFeeApplied": false,
"isMaximumHandlingFeeApplied": false,
"sendAmount": 103.25,
"receiveAmount": 100,
"totalFee": 3.25,
"partnerFee": 3, // Not present if partner fee is not enabled
"handlingFee": 0.25
},
"transferOut": {
"currency": "ETH",
"sendAmount": 0.009582,
"receiveAmount": 0.008582,
"networkFee": 0.001, // Not present if no network fee
"promoCodeValue": {
"amount": 10,
"currency": "EUR"
},
"promoCodeRedeemedAmount": {
"amount": 0.001,
"currency": "ETH"
}
},
"exchangeRate": 10436.23
}
Example response (sell) for
GET /trades/quote/:id/details?transferInMedium=blockchain&transferOutMedium=bank
{
"transferIn": {
"currency": "BTC",
"sendAmount": 0.009582,
"receiveAmount": 0.009582
},
"transferOut": {
"currency": "EUR",
"isMinimumHandlingFeeApplied": false,
"isMaximumHandlingFeeApplied": false,
"sendAmount": 103.25,
"receiveAmount": 100,
"totalFee": 3.25,
"partnerFee": 3, // Not present if partner fee is not enabled
"handlingFee": 0.25
},
"exchangeRate": 10775.41
}
GET https://app-api.coinify.com/trades/quote/:id/details
Endpoint should be used to get an overview of fees and amounts, before creating the trade
Request
The parameters in the request object are as follows:
Parameter | Type | Default | Description |
---|---|---|---|
transferInMedium | String | Required | Transfer medium |
transferOutMedium | String | Required | Transfer medium |
promoCode | String | Optional | Used to validate if promo quote is valid and get new amounts |
Response (buy)
Key | Type | Description |
---|---|---|
transferIn | Object | |
→sendAmount | Number | The amount the end-user has to send to complete the trade. |
→receiveAmount | Number | The amount Coinify system will receive, without the fees. |
→currency | String | The currency denomination of sendAmount and receiveAmount |
→handlingFee | Number | The fee we charge for the payment |
→partnerFee | Number | Partner fee (only present if enabled for partner) |
→totalFee | Number | Handling fee + partner fee, same as the difference between sendAmount and receiveAmount |
→isMinimumHandlingFeeApplied | Boolean | True if handling fee is the same as the minimum fee |
→isMaximumHandlingFeeApplied | Boolean | True if handling fee is the same as the maximum fee |
transferOut | Object | |
→currency | String | |
→sendAmount | Number | The amount before network fee |
→receiveAmount | Number | The amount the trader receives |
→networkFee | Number | Network fee for the transaction |
→promoCodeValue | Object | {amount, currency} of the promo code value in FIAT (only present if promoCode is provided) |
→promoCodeRedeemedAmount | Object | {amount, currency} of the promo code value in Crypto (only present if promoCode is provided) |
exchangeRate | Number | Exchange rate used for this trade |
Response (sell)
Key | Type | Description |
---|---|---|
transferIn | Object | |
→currency | String | |
→sendAmount | Number | The amount the trader has to pay |
→receiveAmount | Number | The amount we receive without the fees |
transferOut | Object | |
→currency | String | |
→sendAmount | Number | The amount we send without the fee |
→receiveAmount | Number | The amount the trader receives after fees are subtracted |
→handlingFee | Number | The fee we charge for the payout |
→partnerFee | Number | Partner fee (only present if enabled for partner) |
→totalFee | Number | Handling fee + partner fee, same as the difference between sendAmount and receiveAmount |
→isMinimumHandlingFeeApplied | Boolean | True if handling fee is the same as the minimum fee |
→isMaximumHandlingFeeApplied | Boolean | True if handling fee is the same as the maximum fee |
exchangeRate | Number | Exchange rate used for this trade |
Create trade
Example request for
POST /trades
, using a valid price quote
{
"priceQuoteId": 123456,
"transferIn": {
"medium": "card"
},
"transferOut": {
"medium": "blockchain",
"details": {
"account": "1A1zP1eP5QGefi2DMPTfTL5SLmv7DivfNa"
}
},
"partnerContext": {
"clientId": 123,
"refId": 12
}
}
Example response for the above request
{
"id": 113475347,
"traderId": 754035,
"traderEmail": "customer@coinify.com",
"state": "awaiting_transfer_in",
"inCurrency": "USD",
"outCurrency": "BTC",
"inAmount": 1000.00,
"outAmountExpected": 2.41526674,
"transferIn": {
"currency": "USD",
"sendAmount": 1000.00,
"receiveAmount": 1000.00,
"medium": "card",
"details": {
"redirectUrl": "https://provider.com/payment/d3aab081-7c5b-4ddb-b28b-c82cc8642a18"
}
},
"transferOut": {
"currency": "BTC",
"medium": "blockchain",
"sendAmount": 2.41526674,
"receiveAmount": 2.41526674,
"details": {
// Trader's bitcoin address that will receive approx. 2.41526674 BTC if trade completes
"account": "1A1zP1eP5QGefi2DMPTfTL5SLmv7DivfNa"
}
},
"quoteExpireTime": "2016-04-01T12:38:19Z",
"updateTime": "2016-04-01T12:27:36Z",
"createTime": "2016-04-01T12:23:19Z"
}
Example request for
POST /trades
(buy bitcoins for bank transfer)
{
"priceQuoteId": 123456,
"transferIn": {
"medium": "bank"
},
"transferOut": {
"medium": "blockchain",
"details": {
"account": "1A1zP1eP5QGefi2DMPTfTL5SLmv7DivfNa"
}
}
}
Example response for the above request
{
"id": 113475347,
"traderId": 754035,
"traderEmail": "customer@coinify.com",
"state": "awaiting_transfer_in",
"inCurrency": "USD",
"outCurrency": "BTC",
"inAmount": 1000.00,
"outAmountExpected": 2.41526674,
"transferIn": {
"currency": "USD",
"sendAmount": 1000.00,
"receiveAmount": 1000.00,
"medium": "bank",
"details": { // Information about where the user should sent the money
"account": {
"currency": "DKK", // Currency of the bank account
"bic": "HIASDMIASD", // Account bic/swift/reg number depending on the type
"number": "1234-34235-3324-2342" // Account number
},
"bank": {
"name": "Bank name", // Name of the bank
"address": { // Address of the bank
"street": "123 Example Street",
"zipcode": "12345",
"city": "Exampleville",
"state": "CA",
"country": "US"
}
},
"holder": {
"name": "John Doe", // Name of the account holder
"address": { // Address of the account holder
"street": "123 Example Street",
"zipcode": "12345",
"city": "Exampleville",
"state": "CA",
"country": "US"
}
}
}
},
"transferOut": {
"currency": "BTC",
"medium": "blockchain",
"sendAmount": 2.41526674,
"receiveAmount": 2.41526674,
"details": {
// Trader's bitcoin address that will receive approx. 2.41526674 BTC if trade completes
"account": "1A1zP1eP5QGefi2DMPTfTL5SLmv7DivfNa"
}
},
"quoteExpireTime": "2016-04-01T12:38:19Z",
"updateTime": "2016-04-01T12:27:36Z",
"createTime": "2016-04-01T12:23:19Z"
}
POST https://app-api.coinify.com/trades
The Create trade endpoint allows a trader to trade crypto for fiat, or vice-versa.
On success, the endpoint returns the newly created trade object.
The endpoint supports the following directions:
- Buy crypto currency with card (
transferIn.medium == "card"
andtransferOut.medium == "blockchain"
) - Buy crypto currency with bank transfer (
transferIn.medium == "bank"
andtransferOut.medium == "blockchain"
) - Sell bitcoins and receive money by bank transfer (
transferIn.medium == "blockchain"
andtransferOut.medium == "bank"
)
Important
Even though it is possible to create trades for all the traders. A trader has to be approved to complete their trades, see Trader section.
You have to provide the ID of a existing price quote (
priceQuoteId
.
Request object
The parameters in the request object are as follows:
Parameter | Type | Default | Description |
---|---|---|---|
priceQuoteId | Integer | Required | Identifier of valid price quote to base the trade on. Passing an invalid price quote will result in an error. |
transferIn | Object | Required | An object describing how Coinify will receive the money for this transfer. |
→medium | String | Required | Transfer medium. |
→details | Object | Optional | Can be used to supply returnUrl for medium=card . Transfer details. |
transferOut | Object | Required | An object describing how Coinify will send the result of the trade to the trader. |
→medium | String | Required | Transfer medium. |
→mediumReceiveAccountId | Integer | Required if medium is bank | Bank account of the trader. |
→ details | Object | Required if medium is blockchain | Transfer details |
partnerContext | JSON | Optional | Partner context which will be returned in trade webhooks. Can be one of object, array, string, number, true, false as defined here https://www.json.org/json-en.html |
HTTP Response code | JSON data |
---|---|
201 Created | Success, object returned, as shown to the right |
401 Unauthorized | Error, access token missing. |
List trades
Example response for
GET /trades
[
{
"id": 113475347,
"traderId": 754035,
"traderEmail": "customer@coinify.com",
"state": "awaiting_transfer_in",
"inCurrency": "USD",
"outCurrency": "BTC",
"inAmount": 1000.00,
"outAmountExpected": 2.41526674,
"transferIn": {
"currency": "USD",
"sendAmount": 1000.00,
"receiveAmount": 1000.00,
"medium": "card",
"details": {
"redirectUrl": "https://provider.com/payment/d3aab081-7c5b-4ddb-b28b-c82cc8642a18"
}
},
"transferOut": {
"currency": "BTC",
"medium": "blockchain",
"sendAmount": 2.41526674,
"receiveAmount": 2.41526674,
"details": {
// Trader's bitcoin address that will receive approx. 2.41526674 BTC if trade completes
"account": "1A1zP1eP5QGefi2DMPTfTL5SLmv7DivfNa"
}
},
"quoteExpireTime": "2016-04-01T12:38:19Z",
"updateTime": "2016-04-01T12:27:36Z",
"createTime": "2016-04-01T12:23:19Z"
},
{
// Next trade...
}
]
GET https://app-api.coinify.com/trades
Response
This endpoint lists all trade objects belonging to the authorized trader.
The trades are ordered by id
(highest first).
HTTP Response code | JSON data |
---|---|
200 OK | Success, response as shown to the right. |
400 Bad request | Error interpreting the request. |
404 Not found | Error, trades that belong to the provided trader were not found. |
Query parameters
Parameter | Type | Description |
---|---|---|
limit | String | Limit used for pagination (default: 100, max: 100) |
offset | String | Offset used for pagination (default: 0) |
trade_state | String | Return only trades in specific state(s) (optional: will include all states by default) |
Get trade information
Example response for
GET /trades/113475347
{
"id": 113475347,
"traderId": 754035,
"traderEmail": "customer@coinify.com",
"state": "awaiting_transfer_in",
"inCurrency": "USD",
"outCurrency": "BTC",
"inAmount": 1000.00,
"outAmountExpected": 2.41526674,
"transferIn": {
"currency": "USD",
"sendAmount": 1000.00,
"receiveAmount": 1000.00,
"medium": "card",
"details": {
"redirectUrl": "https://provider.com/payment/d3aab081-7c5b-4ddb-b28b-c82cc8642a18"
}
},
"transferOut": {
"currency": "BTC",
"medium": "blockchain",
"sendAmount": 2.41526674,
"receiveAmount": 2.41526674,
"details": {
// Trader's bitcoin address that will receive approx. 2.41526674 BTC if trade completes
"account": "1A1zP1eP5QGefi2DMPTfTL5SLmv7DivfNa"
}
},
"quoteExpireTime": "2016-04-01T12:38:19Z",
"updateTime": "2016-04-01T12:27:36Z",
"createTime": "2016-04-01T12:23:19Z"
}
GET https://app-api.coinify.com/trades/<id>
This endpoint returns a trade object for the trade with ID <id>
.
Response
See trade object.
HTTP Response code | JSON data |
---|---|
200 OK | Success, response as shown to the right. |
400 Bad request | Error interpreting the request. |
404 Not found | Error, trade is not found (no trade with such ID exists or no trade with such ID that belongs to the provided trader). |
Cancel trade
Example response for
PATCH /trades/113475347/cancel
{
"id": 113475347,
"traderId": 754035,
"traderEmail": "customer@coinify.com",
// State is now "cancelled"
"state": "cancelled",
"inCurrency": "USD",
"outCurrency": "BTC",
"inAmount": 1000.00,
"outAmountExpected": 2.41526674,
"transferIn": {
"currency": "USD",
"sendAmount": 1000.00,
"receiveAmount": 1000.00,
"medium": "card",
"details": {
"redirectUrl": "https://provider.com/payment/d3aab081-7c5b-4ddb-b28b-c82cc8642a18"
}
},
"transferOut": {
"currency": "BTC",
"medium": "blockchain",
"sendAmount": 2.41526674,
"receiveAmount": 2.41526674,
"details": {
"account": "1A1zP1eP5QGefi2DMPTfTL5SLmv7DivfNa"
}
},
"updateTime": "2016-04-01T12:34:37Z",
"createTime": "2016-04-01T12:23:19Z"
}
PATCH https://app-api.coinify.com/trades/<id>/cancel
This endpoint cancels the trade in the awaiting_transfer_in
state and returns a trade object for the cancelled trade.
Request object
This endpoint requires no request body
Response
See trade object.
HTTP Response code | JSON data |
---|---|
200 OK | Success, response as shown to the right. |
400 Bad request | Error trade not cancellable. |
404 Not found | Error, trade is not found (no trade with such ID exists or no trade with such ID that belongs to the provided trader). |
Testing
This section explains how you can complete trades that you create in the Sandbox environment.
Before being able to complete the trades, make sure you have succesfully completed these actions and provided the following mandatory trader information:
- Verify trade account email
- Complete identification process or Skip the identification process in Sandbox
- Provide trader's address
- Provide Source of Funds
- Provide PEP (Politically Exposed Person)
This section contains the following endpoints that allow you to test the full end-to-end trade flow:
- Force complete trade
- Complete trade with test card
- Complete trade with a fake bank transaction
- Skip KYC in Sandbox
Force complete trade
Example request for
POST /trades/113475347/test/complete-trade
{
"details": {
"account": "1A1zP1eP5QGefi2DMPTfTL5SLmv7DivfNa"
},
"amount": 200.00, // Transfer out amount of the fake transaction
"currency": "EUR" // Transfer out currency of the amount
}
POST https://app-api.sandbox.coinify.com/trades/<id>/test/complete-trade
This endpoint will complete a newly created trade which is in the awaiting_transfer_in
state.
Provide the ID of the trade in the <id>
path parameter to complete the specific.
Request object
The parameters in the request object are optional and are use to validate the trade if they are sent:
Parameter | Type | Default | Description |
---|---|---|---|
amount | Float | (Optional) | Transfer out receive amount of the trade. |
currency | String | (Optional) | Currency of the amount. |
details | Medium details | (Optional) | Information relevant for this medium |
Details object
In the details you must specify the account
of the transfer out.
If the referenced trade is not in the awaiting_transfer_in
state, or if the amount is not the same as the stated in the transfer, the endpoint will return a 500 Internal Error
error.
Response
This endpoint returns 204 No Content
if the trade is completed
successfully.
HTTP Response code | JSON data |
---|---|
204 No Content | Success, empty response. |
400 Bad request | Error interpreting the request. |
500 Server error | Error error has occurred parsing the data. |
Complete trade with a test Card payment
Use the following test cards to complete a Buy trade via card payment.
Card Number | Name on card | Expected Result |
---|---|---|
4761344136141390 | FL-BRW1 | Card payment Successful |
4000000000001000 | Any name | Card payment Successful Use if the above card doesn't work |
4761344136141390 | CL-BRW1 | Challenge flow (3DS) |
4000164166749263 | FL-BRW1 | Do not honour |
5001638548736201 | FL-BRW1 | Declined |
4000128449498204 | ERR-BRW1 | Error |
Complete trade with a fake bank transaction
Example request for
POST /trades/113475347/test/bank-transfer
{
"sendAmount": 200.00, // Amount of the fake transaction
"currency": "EUR" // Currency of the amount
}
POST https://app-api.sandbox.coinify.com/trades/<id>/test/bank-transfer
This endpoint creates a fake bank transfer for the trade with ID <id>
and processes the trade as if a real bank transfer had occurred.
Request object
The parameters in the request object are as follows:
Parameter | Type | Default | Description |
---|---|---|---|
sendAmount | Integer | Required | Amount sent from the trader's bank account. This corresponds to the transferIn.sendAmount of the trade. |
currency | String | Required | Currency of the amount. |
awaiting_transfer_in
state or the transferIn.medium
is not bank
, the endpoint will return a 400 Bad Request
error.
Response
This endpoint returns 204 No Content
if the fake bank transfer creation succeeded.
HTTP Response code | JSON data |
---|---|
204 No Content | Success, empty response. |
400 Bad request | Error interpreting the request. |
500 Server error | Error error has occurred parsing the data. |
Complete/Skip KYC
In the sandbox environment it is possible to automatically approve an identification-attempt by including state: 'approved'
in the request object.
See example on the right or follow a more detailed explanation in this article.
Example of request to approve identification attempt
{
"returnUrl": "https://mypage.com/ida_complete",
"state": "approved"
}